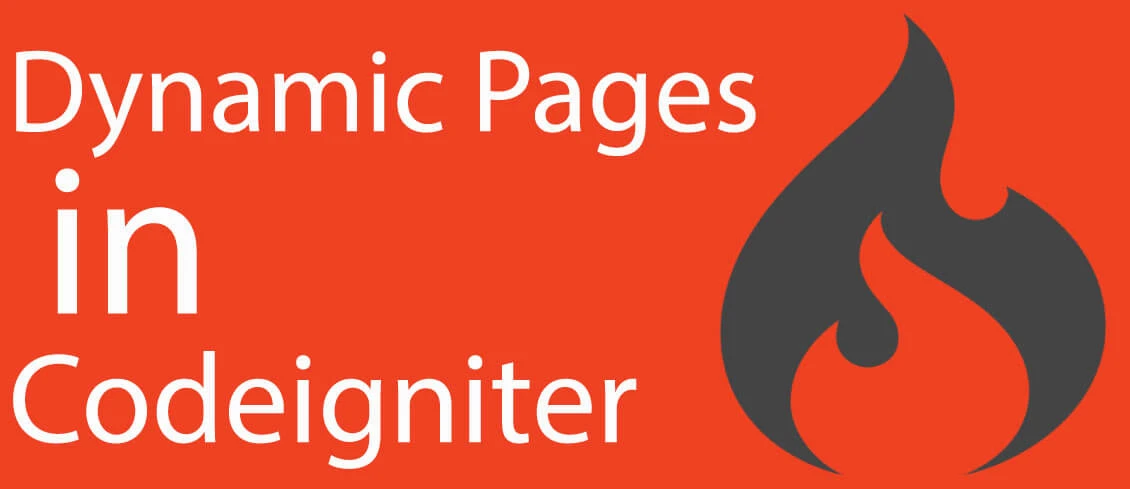
- Views: 5.0K
- Category: Codeigniter
- Published at: 07 Apr, 2020
- Updated at: 12 Aug, 2023
Create dynamic pages in Codeigniter like WordPress
What are dynamic pages? This is a question, and the answer is straightforward; if you ever used any CMS system like WordPress or Joomla, you better understand the pages. Let's suppose you have a domain name xyz.com and here is another fuel xyz.com/your-page-slug-here so this is the SEO-friendly URL. If you want to rank your website on Google's first page, you have to create/manage your URLs like that.
But how can you achieve this task in Codeigniter? We know the Codeigniter URL structure xyz.com/controller/method/parameters, so after xyz.com, we always use the controller name like Home, etc. but when you take about the dynamic pages, you may have multiple pages, maybe 100. Possibly on a thousand, you have the solution which means you have to create 100 or thousands of controllers/pages manually, so I think it's hard for you to create the controllers. Let me take another example. Let's suppose you are designing the system for your client, and your clients want to add the page. Then, finally, your client can't change/create the controller.
Creating the dynamic pages in CodeIgniter is a difficult task because you have to manage the CodeIgniter routes; in WordPress, we have a text editor to create/manage the pages. Let's suppose you need the same page system in Codeigniter.
So I can achieve this goal, I am going to explain how can you create dynamic pages in Codeigniter like WordPress.
Before starting, you have to use remove the index.php from the URL in Codeigniter, and set the $config['base_url'] = 'http://localhost/aci/'; aci is the folder name where I keep all my Codeigniter files.
Open your autoload.php file and set some libraries, helpers, and models to upload.
$autoload['libraries'] = array('database','session','form_validation');
$autoload['helper'] = array('url','custom_helper','file','form','string');
$autoload['model'] = array('modHome');
Step 1: Create the home controller named Home and use this code to create the pages.
class Home extends CI_Controller
{
public function index()
{
$this->load->view('headFoot/header');
$this->load->view('headFoot/navbar');
$this->load->view('home/home');
$this->load->view('headFoot/footer');
$this->load->view('headFoot/htmlClose');
}
public function newPage()
{
$this->load->view('headFoot/header');
$this->load->view('headFoot/navbar');
$this->load->view('home/newPage');
$this->load->view('headFoot/footer');
$this->load->view('headFoot/htmlClose');
}
public function createPage()
{
$this->form_validation->set_rules('pageName','Page Name','required');
$this->form_validation->set_rules('pageText','Page Content','required');
if ($this->form_validation->run() == false) {
$this->newPage();
}
else {
$data['p_name'] = $this->input->post('pageName', true);
$data['p_description'] = $this->input->post('pageText', true);
$data['p_date'] = date('Y-m-d h:i:sa');
$data['p_slug'] = $this->seoURL($data['p_name']);
$checkPage = $this->modHome->checkPage($data);
if ($checkPage->num_rows() > 0) {
customFlash('You have already created the page.','alert-warning','home/createPage');
}
else{
$addPage = $this->modHome->addPage($data);
if ($addPage) {
$this->save_routes();
customFlash('You have successfully created the page','alert-success','home/createPage');
}
else{
customFlash('Something went wrong please try again','alert-danger','home/createPage');
}
}
}
}
private function seoURL($text)
{
$text = strtolower(htmlentities($text));
$text = str_replace(" ", "-", $text);
return $text;
}
private function save_routes(){
$routes = $this->modHome->get_all_routes();
$data = array();
if (!empty($routes)) {
$data[] = '<?php ';
foreach ($routes as $route) {
$data[] = '$route[\''.$route['p_slug'].'\'] = \''.'pages'.'/'.'index/'.$route['p_id'].'\';';
}
$output = implode("\n",$data);
write_file(APPPATH.'cache/routes.php',$output);
}
else{
echo 'else here';
}
}
}
In the above controller, I am breaking the HTML code into multiple part/views
Create a database named aci and create a table called pages
CREATE TABLE `pages` (
`p_id` int(11) NOT NULL,
`p_name` varchar(200) NOT NULL,
`p_description` text NOT NULL,
`p_date` datetime NOT NULL,
`p_status` int(11) DEFAULT '1',
`p_slug` varchar(250) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
ALTER TABLE `pages`
ADD PRIMARY KEY (`p_id`);
ALTER TABLE `pages`
MODIFY `p_id` int(11) NOT NULL AUTO_INCREMENT;
COMMIT;
Step 2: Go to the application/cache folder and create a new PHP file named routes.php
Step 3: Now, Go to the application/config/routes.php and spend the file and add your ourtes.php, which you have just created in step 2.
require_once APPPATH . 'cache/routes.php';
Step 4: Create the model and use this code to create the dynamic pages in Codeigniter.
<?php
class ModHome extends CI_Model
{
public function checkPage($data)
{
return $this->db->get_where('pages',array('p_name'=>$data['p_name']));
}
public function addPage($data)
{
return $this->db->insert('pages',$data);
}
public function get_all_routes()
{
return $this->db->get_where('pages',array('p_status'=>1))->result_array();
}
public function checkbyPageId($url)
{
return
$this->db->get_where('pages',array('p_id'=>$url))->result_array();
}
public function methodNme($data)
{
return $this->db->insert_batch('tableName',$data);
}
}
Step 5: Create a view named newPage and keep the home directory view and use the below code.
<div class="container martop">
<div class="row">
<div class="col-md-6">
<?php echo validation_errors(); ?>
<?php echo checkFlash();?>
<form action="<?php echo site_url('home/createPage')?>" method="post">
<div class="form-group">
<input type="text" class="form-control" name="pageName" placeholder="Enter your page name">
</div>
<div class="form-group">
<textarea name="pageText" class="form-control" cols="10" rows="10"></textarea>
</div>
<div class="form-group">
<button type="submit" class="btn btn-primary">Create the page</button>
</div>
</form>
</div>
</div>
</div>
Now access your page URL
http://localhost/aci/shekztech-page-here
I am using the Bootstrap classes in my HTML code because I have added the CSS and JS files in Codeigniter. if you want to add CSS and JS files in Codeigniter 4
Make sure I have the checkFlash() method in the code because I have created the custom helper and used the method/function from it.
Download the source code
https://www.youtube.com/watch?v=0wFgenn4RXU
0 Comment(s)