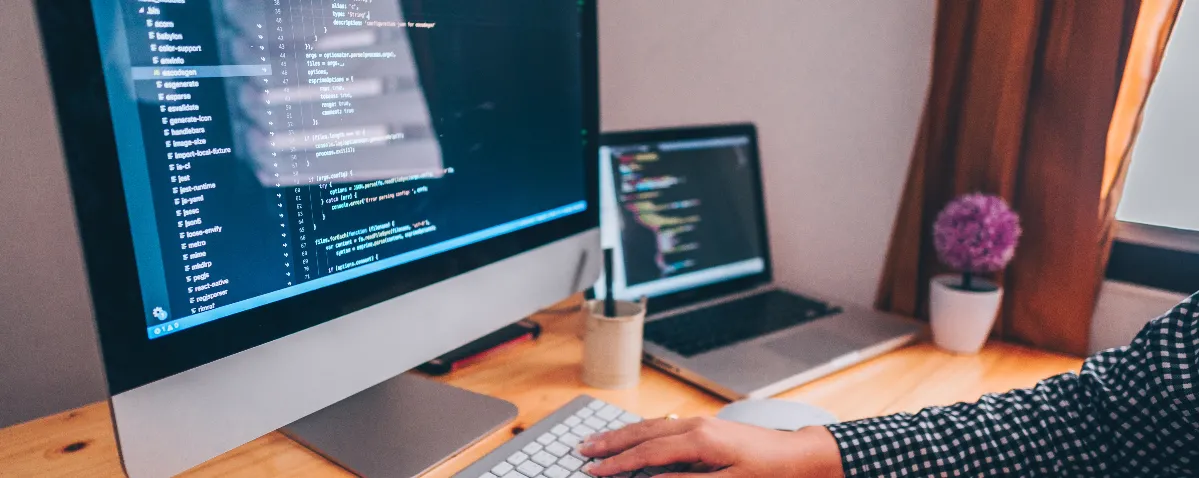
- Views: 4.0K
- Category: Codeigniter
- Published at: 02 Feb, 2017
- Updated at: 18 Aug, 2023
How to create pagination using codeigniter
How to create pagination using codeigniter
What is Pagination..? If you have a lot of content to show on a single page, it's impossible to explain thousands of blogs/products on a single page, so you are ultimately forced to divide them into multiple pages. You can view the pagination in Codeigniter 4.
If you have a lot of content to show on a single page, it's impossible to show thousands of blogs/products on a single page, so you are ultimately forced to divide them into multiple pages. This process is called Pagination.
If you have ever created Pagination using PHP, it is hard to manage using core PHP/custom PHP. Still, using CodeIgniter, creating Pagination for your website/web application is straightforward.
Today I will show you how to create Pagination in ci.
Create a table named users and insert some content; you know very well how to create a table and insert some content :D
my_users
id | fname | lname | gender | |
1 | Shakzee | Ahmed | info@shakzee.com | ale |
2 | Hassan | jawaid | hassan@hotmail.com | male |
3 | Sidra | Ali | sidra@hotmail.com | female |
Create a controller named users and a function myusers.
Note: we are creating Pagination for myusers function, not the main controller/index
<?php
class Users extends CI_Controller
{
public function index(){
//this is you index function.
}
public function myusers()
{
$this->load->model('model_users');
$config = array();
$config['full_tag_open'] = '';
$config['full_tag_close'] = '';
$config['first_link'] = FALSE;
$config['last_link'] = FALSE;
$config['first_tag_open'] = '';
$config['first_tag_close'] = '';
$config['last_tag_open'] = '';
$config['last_tag_close'] = '';
$config['next_link'] = 'Next';
$config['next_tag_open'] = '';
$config['next_tag_close'] = '';
$config['prev_link'] = 'Prev';
$config['prev_tag_open'] = '';
$config['prev_tag_close'] = '';
$config['cur_tag_open'] = '';
$config['cur_tag_close'] = '';
$config['num_tag_open'] = '';
$config['num_tag_close'] = '';
$config["base_url"] = base_url().'users/myusers';
$q_sortas = null;
$tot = $this->model_users->get_all_users();
$config["total_rows"] = $tot->num_rows();
$config["per_page"] = 5; //showing result per page
$config["uri_segment"] = 3;
$this->load->library('pagination'); //loading the codeigniter library
$this->pagination->initialize($config); //here we initializing our settings
$page = ($this->uri->segment(3)) ? $this->uri->segment(3) : 0;
$data["users"] = $this->model_users->fatch_all_users($config["per_page"], $page);
$data["links"] = $this->pagination->create_links(); //pagination links
$data['title'] = "Demo | Pagination in codeigniter | shakzee";
$this->load->view('pagination',$data);
}
}
$config["total_rows"]
means we are counting total row/records in the table.
You always receive 5 records because $config["per_page"] = 5;
Now create a model named Model_users and two functions.
<?php
class Model_users extends CI_Model
{
public function get_all_users()
{
return $this->db->select('*')
->from('my_users')
->get();
}
public function fatch_all_users($limit, $start)
{
$this->db->limit($limit, $start);
$query = $this->db->select('*')
->from('my_users')
->get();
if ($query->num_rows() > 0)
{
foreach ($query->result() as $row)
{
$data[] = $row;
}
return $data;
}
return false;
}
}//Model_users ends here
Now create a view named Pagination.
<html>
<head>
<title><?php echo $title?></title>
</head>
<body>
Pagination in Codeigniter
<?php if ($users): ?>
<?php foreach($users as $myuser): ?>
<?php echo $myuser->id;?>
<?php echo $myuser->fname;?>
<?php echo $myuser->lname;?>
<?php echo $myuser->email;?>
<?php echo $myuser->gender;?>
<?php endforeach; ?>
<?php echo $links;?>
<?php else: ?>
<?php endif; ?>
</body>
</html>
If you want to set pagination in your main controller/index function in CodeIgniter just add one thing and change something :) it is easy just go to your application/config/routes.phpadd this line at the end of script $route['users/(:num)'] = 'users/index/$1';
Now change uri_segment from 3 to 2 and baser_url() from users/myusers to users
Now your code looks like this.
public function index(){
$this->load->model('model_users');
$config = array();
$config['full_tag_open'] = '';
$config['full_tag_close'] = '';
$config['first_link'] = FALSE;
$config['last_link'] = FALSE;
$config['first_tag_open'] = '';
$config['first_tag_close'] = '';
$config['last_tag_open'] = '';
$config['last_tag_close'] = '';
$config['next_link'] = 'Next';
$config['next_tag_open'] = '';
$config['next_tag_close'] = '';
$config['prev_link'] = 'Prev';
$config['prev_tag_open'] = '';
$config['prev_tag_close'] = '';
$config['cur_tag_open'] = '';
$config['cur_tag_close'] = '';
$config['num_tag_open'] = '';
$config['num_tag_close'] = '';
$config["base_url"] = base_url().'users';
$tot = $this->model_users->get_all_users();
$config["total_rows"] = $tot->num_rows();
$config["per_page"] = 5;
$config["uri_segment"] = 2;
$this->load->library('pagination');
$this->pagination->initialize($config);
$page = ($this->uri->segment(2)) ? $this->uri->segment(2) : 0;
$data["users"] = $this->model_users->fatch_all_users($config["per_page"], $page);
$data["links"] = $this->pagination->create_links();
$data['title'] = " Demo | Pagination in codeigniter | shakzee";
$this->load->view('pagination',$data);
}
https://www.youtube.com/watch?v=mSNcs-O-MqM
- Tag
- Ci
- pagination
0 Comment(s)