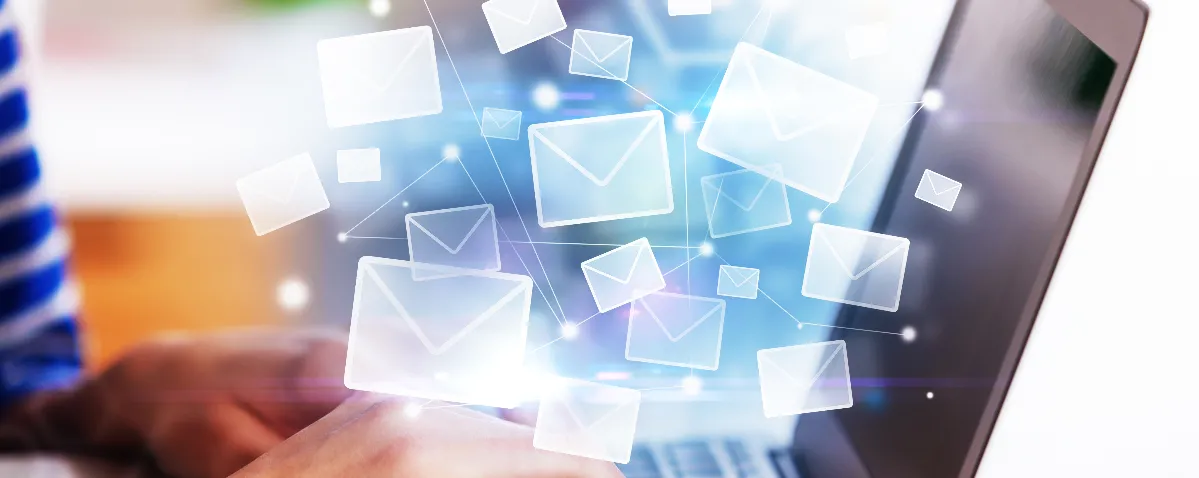
- Views: 4.6K
- Category: Codeigniter
- Published at: 02 Feb, 2017
- Updated at: 18 Aug, 2023
How to send emails from your servers using codeigniter
How to send emails from your servers using Codeigniter
If you are a web developer, you continually design a system to send emails from your system/website. How to send email in Codeigniter 4.
In other words, you still send emails to the users; when a user is creating an account on your system/website, you always verify the email address provided by the user to send an email address from your system.
If you are learning Codeigniter or don't know how to send emails from your server to the user, how can you send emails from your server? Today I will show you how to send emails from your server using CI.
Create a controller Email send and keep it in your controller folder in CodeIgniter.
NOTE: Before using the email class in CodeIgniter, you must load it.
<?php
class Emailsend extends CI_Controller
{
$this->load->library('email')
$config = array(
'useragent' => 'CodeIgniter',
'protocol' => 'mail',
'mailpath' => '/usr/sbin/sendmail',
'smtp_host' => 'localhost',
'smtp_user' => 'info@xyz.com', //change it to yours (this is your email)
'smtp_pass' => 'abcdef', //change it to yours (this is your password)
'smtp_port' => 25,
'smtp_timeout' => 55,
'wordwrap' => TRUE,
'wrapchars' => 76,
'mailtype' => 'html',
'charset' => 'utf-8',
'validate' => FALSE,
'priority' => 3,
'crlf' => "\r\n",
'newline' => "\r\n",
'bcc_batch_mode' => FALSE,
'bcc_batch_size' => 200,
);
$messg = "Your message here";
$this->load->library('email', $config);
$this->email->set_newline("\r\n");
$this->email->from('info@xyz.com','Shakzee');
$this->email->to("info@shakzee.com");// change it to yours
$this->email->subject('here is your subject');
$this->email->message($messg);
$this->email->set_mailtype('html');
$this->email->send();
}
This function $this->email->send(); send email to info@shekztech.com but write $this->db->send(); function in if statement so you can show a message.
Now your code looks like this:
<?php
class Emailsend extends CI_Controller
{
$this->load->library('email')
$config = array(
'useragent' => 'CodeIgniter',
'protocol' => 'mail',
'mailpath' => '/usr/sbin/sendmail',
'smtp_host' => 'localhost',
'smtp_user' => 'info@xyz.com', //change it to yours (this is your email)
'smtp_pass' => 'abcdef', //change it to yours (this is your password)
'smtp_port' => 25,
'smtp_timeout' => 55,
'wordwrap' => TRUE,
'wrapchars' => 76,
'mailtype' => 'html',
'charset' => 'utf-8',
'validate' => FALSE,
'priority' => 3,
'crlf' => "\r\n",
'newline' => "\r\n",
'bcc_batch_mode' => FALSE,
'bcc_batch_size' => 200,
);
$messg = "Your message here";
$this->load->library('email', $config);
$this->email->set_newline("\r\n");
$this->email->from('info@xyz.com','Shakzee');
$this->email->to("info@shakzee.com");// change it to yours
$this->email->subject('here is your subject');
$this->email->message($messg);
$this->email->set_mailtype('html');
$this->email->send();
if($this->email->send())
{
echo "You have been successfuy sent an email";
}
else
{
echo "Here is your custom error message in case of faluire";
}
}
?>
If you want to send a view with your email in CodeIgniter, it's simple; create a view email_view and write your code in that view; it's a normal view, but you must pass third parameters TRUE.
$this->load->view("email_view",$data,TRUE);
Now your code looks like this:
<?php
class Emailsend extends CI_Controller
{
$this->load->library('email')
$config = array(
'useragent' => 'CodeIgniter',
'protocol' => 'mail',
'mailpath' => '/usr/sbin/sendmail',
'smtp_host' => 'localhost',
'smtp_user' => 'info@xyz.com', //change it to yours (this is your email)
'smtp_pass' => 'abcdef', //change it to yours (this is your password)
'smtp_port' => 25,
'smtp_timeout' => 55,
'wordwrap' => TRUE,
'wrapchars' => 76,
'mailtype' => 'html',
'charset' => 'utf-8',
'validate' => FALSE,
'priority' => 3,
'crlf' => "\r\n",
'newline' => "\r\n",
'bcc_batch_mode' => FALSE,
'bcc_batch_size' => 200,
);
$messg = $this->load->view("email_view",$data,TRUE);
$this->load->library('email', $config);
$this->email->set_newline("\r\n");
$this->email->from('info@xyz.com','Shakzee');
$this->email->to("info@shakzee.com");// change it to yours
$this->email->subject('here is your subject');
$this->email->message($messg);
$this->email->set_mailtype('html');
if($this->email->send())
{
echo "You have been successfuy sent an email";
}
else
{
echo "Here is your custom error message in case of failuer";
}
}
?>
https://www.youtube.com/watch?v=QdudJGyOapU
0 Comment(s)