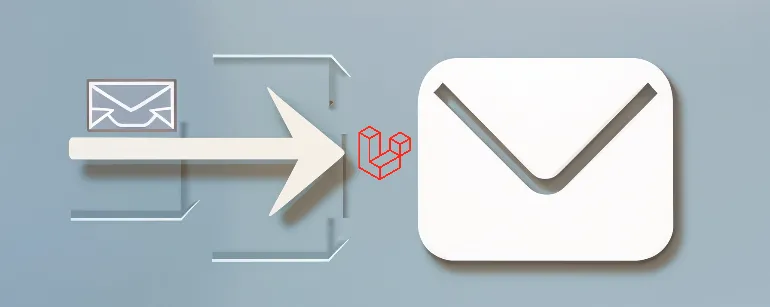
- Views: 742
- Category: Laravel
- Published at: 02 Sep, 2023
- Updated at: 03 Sep, 2023
How to Send Emails with Queues in Laravel 10
Sending emails is a critical functionality for many web applications. However, sending emails directly within your application's main process can slow it down and negatively impact the user experience. Thankfully, Laravel provides an elegant solution to this problem by allowing you to send emails asynchronously using queues. This blog post will guide you step-by-step on how to send emails using queues in Laravel 10.
Why Use Queues/Jobs for Sending Emails?
Utilizing queues for sending emails offers several advantages. Primarily, it improves application performance and responsiveness. When a user performs an action that triggers an email, sending it immediately could slow down the application. With queues, the email task is offloaded to a separate process, allowing your application to continue running smoothly. This results in a more responsive and user-friendly experience.
Step 1: Define the Emails in Laravel
Firstly, you'll need to define the email class in Laravel. You can use Laravel's Artisan command to generate a new Mailable class called NewsletterEmail.
php artisan make:mail NewsletterEmail
Below is a sample code for the NewsletterEmail class, which uses Laravel's Mailable features to define the content and other aspects of the email.
// App/Mail/NewsletterEmail.php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Mail\Mailables\Content;
use Illuminate\Mail\Mailables\Envelope;
use Illuminate\Queue\SerializesModels;
class NewsletterEmail extends Mailable
{
use Queueable, SerializesModels;
protected $unsubscribeToken;
public function __construct($unsubscribeToken)
{
$this->unsubscribeToken = $unsubscribeToken;
}
public function envelope(): Envelope
{
return new Envelope(
subject: 'Newsletter Email',
);
}
public function content(): Content
{
return new Content(
markdown: 'emails.newsletter',
with: [
'unsubscribeToken' => $this->unsubscribeToken,
],
);
}
public function attachments(): array
{
return [];
}
}
The NewsletterEmail class uses Laravel's Mailable class to define the email content, subject, and even possible attachments.
Here is the view emails.newsletter
@component('mail::message')
# Hello!
Thank you for subscribing to our newsletter. You'll now be the first to know about our new products, updates, and more!
@component('mail::button', ['url' => url('/')])
Visit our website
@endcomponent
If you did not subscribe to this newsletter and think this is a mistake, click the button below to unsubscribe.
@component('mail::button', ['url' => url('/unsubscribe'.'/'.$unsubscribeToken)])
Unsubscribe
@endcomponent
Thanks,<br>
{{ config('app.name') }}
@endcomponent
Step 2: Define Jobs in Laravel
Next, you'll need to create a job that will handle the email sending logic. Use the Artisan command to create this job.
php artisan make:job SendNewsletterEmail
Here is how you can define the SendNewsletterEmail job:
// App/Jobs/SendNewsletterEmail.php
namespace App\Jobs;
use App\Mail\NewsletterEmail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use Illuminate\Support\Facades\Mail;
class SendNewsletterEmail implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $email;
protected $unsubscribeToken;
public function __construct($email, $unsubscribeToken)
{
$this->email = $email;
$this->unsubscribeToken = $unsubscribeToken;
}
public function handle(): void
{
Mail::to($this->email)->send(new NewsletterEmail($this->unsubscribeToken));
}
}
In this job, we implement ShouldQueue to indicate that this job should be queued. The handle() method is where we actually send the email using the Mail facade.
Step 3: Dispatch the Email
Finally, you'll need to dispatch the job to send the email. Let's create a method called myMethod to do this:
// In your relevant controller or service class
use Illuminate\Support\Str;
use App\Jobs\SendNewsletterEmail;
public function myMethod(){
$unsubscribeToken = Str::random(40);
$email = 'info@shekztech.com';
dispatch(new SendNewsletterEmail($email, $unsubscribeToken));
}
In the myMethod function, we generate a random unsubscribe token, specify the recipient email, and then dispatch the SendNewsletterEmail job.
Conclusion
Sending emails using queues in Laravel is a robust way to improve your application's performance. This blog post has guided you through the complete setup, from defining the email and job classes to dispatching the email using a queue. By following these steps, you can ensure a faster, more efficient, and user-friendly application.
- Tag
- Laravel 10
- Queues
- Emails
0 Comment(s)