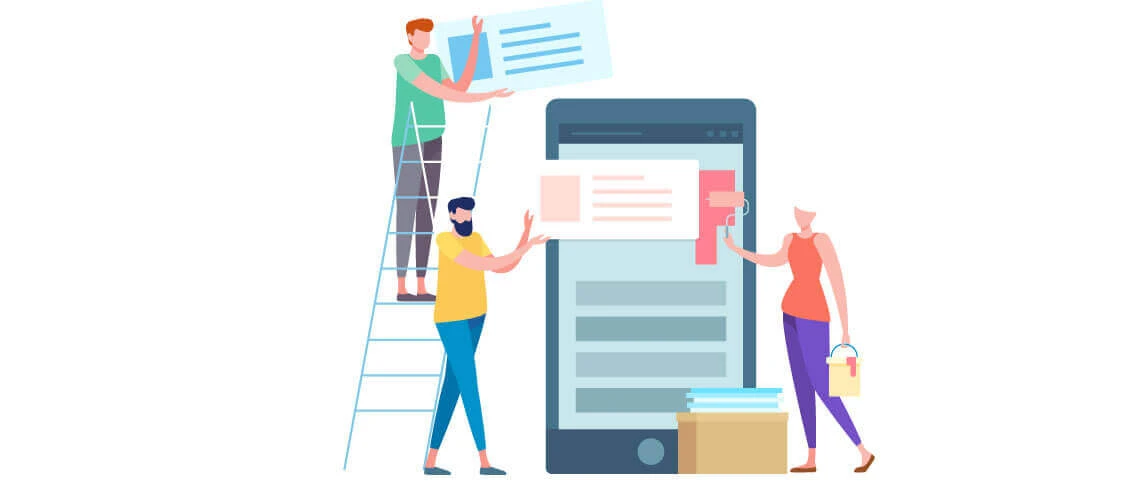
- Views: 4.0K
- Category: Codeigniter
- Published at: 14 Mar, 2018
- Updated at: 12 Aug, 2023
Why you need to break your HTML Content into multiple views in codeigniter
Why do you need to break HTML Content into multiple views?
If you are working on Any MVC Framework like Laravel or Codeigniter, you create your HTML content view, especially in CodeIgniter. As a programmer, you must reduce the code and improve your web application/website's performance. We always load our view inside any controller or method, and if you have many plugins on your site, then it's hard to manage the website performance because we know that if we have 30 plugins, we have 30 CSS and JS files. You will attach 60 CSS and JS files, and then definitely, it will decrease your performance; this is the main problem if you consider the performance.
Here is your home controller.
<?php class Home extends CI_Controller
{
function __construct()
{
parent::__construct(); $this->clear_cache();
}
public function index() {
$this->load->view('home');
}
} ?>
Here is the home.php view
<!DOCTYPE html>
<html>
<head>
<title>Title</title> Your all css files here
</head>
<body>
Navigation bar here your content here your footer here your all js files here
</body>
</html>
Consider the above code; if you are coding like that with a single view, this is not a good practice because it's just a unique method; if you have multiple controllers and multiple ways, it's hard to manage your code. Now you have to ask why..? because when you update the navigation section from a specific method, you must update the other method. How can you update the navigation bar from all the methods if you have thousands of ways?
The other problem is your plugins; if you are following the above approach, you add all your CSS and JS files; okay, it's okay, but what's wrong with this approach? Suppose you have five plugins, and each plugin you will add in a specific method; you have only one choice you have to add all your CSS and JS files in your view.
<!DOCTYPE html>
<html>
<head>
<title>Title</title>
<link type="text/css" rel="" href="plugin1">
<link type="text/css" rel="" href="plugin2">
<link type="text/css" rel="" href="plugin3">
<link type="text/css" rel="" href="plugin4">
<link type="text/css" rel="" href="plugin5">
</head>
<body>
<script type="text/javascript" src="plugin1"></script>
<script type="text/javascript" src="plugin2"></script>
<script type="text/javascript" src="plugin3"></script>
<script type="text/javascript" src="plugin4"></script>
<script type="text/javascript" src="plugin5"></script>
</body>
</html>
Suppose you have 100 plugins and want to add all your plugins in a specific method, i.e., about us, contact us, so this is not good for your site these are main reasons or problems but what about the solution....?
Before solving the problem, survey the site, i.e. (wwww.shekztech.com) or any website. They have a header, navigation bar, content section, and footer section; these are the current sections available on each site.
Now, what do you have to do..? you need to break your HTML content into multiple views, i.e., for the navigation bar, create your look for navigation and a header, and footer, and load these views in your every method if you have a unique look. You are loading that view in all your processes. Whenever you want to update any section from what you need, you need to open that view, i.e., the navigation bar, and update it. It's simply because you now have a single view for your specific section.
Now create these views inside your view directory header, CSS, navbar, home, footer, chtml.
Now open your header.php view and add code to your view.
<!DOCTYPE html>
<html lang="en">
<head>
<meta name="msvalidate.01" content="1D36512A9BDA241273B640F61C6222BF" />
<title>
</title>
Now open your css.php view, and below the code, make sure you have to add those CSS files required for all methods, i.e., bootstrap and your custom style.
<link href="<?php echo base_url('bootstrap') ?>" rel="stylesheet">
<link href="<?php echo base_url('custom sytle') ?>" rel="stylesheet">
Open your navbar.php view and add your navigation code.
</head>
<body>
navigation bar here
And now, open your home.php view and add your main content related to your method.
<h6>content </h6>
<h6>content </h6>
Open your footer.php view and add your footer section here; make sure to add your jquery and those js files required for all methods, i.e., custom.js file.
<!-- Jquery --> <script src="jquery"></script>
<script src="customjsfile"></script>
Finally, open your chtml.php view and close your HTML and body.
</body>
</html>
Now your code looks like this.
public function yourMethod(){
$this->load->view('header');
$this->load->view('css');
$this->load->view('navbar');
$this->load->view('home',$data);
$this->load->view('footer');
$this->load->view('chtml');
}
What about your extra plugin, which you want to add to your specific method? It's simple, load a new view below the CSS view and add your additional js files below the footer; check the below code.
public function yourSpecifiMethod(){
$this->load->view('header');
$this->load->view('css');
$this->load->view('extra css plugin');//specific plugin
$this->load->view('navbar');//Your are closing the head tag here $this->load->view('contentRelatedWithYourMethod',$data);
$this->load->view('footer');
$this->load->view('extra js plugin');//specific plugin
$this->load->view('chtml');// your are closing the body and html tag here
}
https://www.youtube.com/watch?v=GEZQVL8791w
- Tag
- Break HTML
- Codeigniter
- Ci
0 Comment(s)